Coding is underneath nearly everything—from automation systems that drive our manufacturing plants to recommendation systems getting us addicted to Facebook. Coding is now the ultimate solution to problems. It’s fascinating and inspiring: who hasn’t seen the Iron-Man movies, and didn’t dream of creating a Jarvis program of his own?
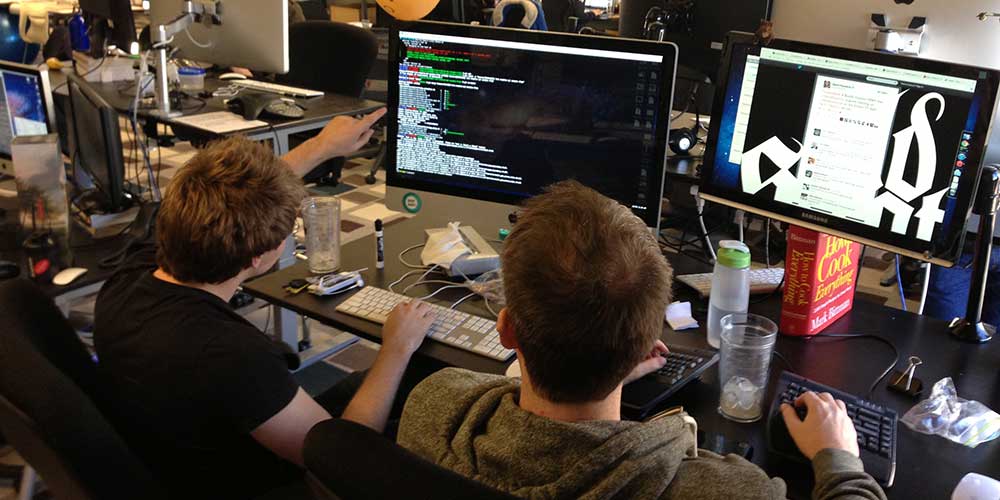
It’s engaging to think that complex products that are used by millions can be created out of thin air by hackers typing code. But learning to become such an able hacker is no easy task.
In my personal quest to learn to code, I was first directed to Competitive Coding. For those who don’t know, that’s the sport where you’re given a problem, and submit code for solving the problem to a website (such as SPOJ). The site checks if your code solves the problem correctly. If so, you get a green tick next to the programming challenge you solved.
In my rush to get as many green ticks as I could, I’d code very roughly. Getting the code accepted by the judge program and satisfying my ego were the only goals. I had no concern for software quality. And that was fine—for I was learning the stuff that comes up in coding interviews: data structures and algorithms.
For a first contact with algorithms, data structures and problem solving strategies, see the book Computer Science Distilled. It’s a slim intro to computer science that includes many basic principles every programmer should know, in a simple way. Check it out!
I was also studying computer science at college. A few semesters later, I was given the task to create a large software project. Trying to write a program containing thousands of lines of code made me realize I didn’t actually knew coding, at all. I didn’t know how to think of abstraction in multiple layers, how to properly name variables (they always used to be x, y, z), how to break down a problem into independent smaller problems, so that the code is testable. My addiction to those green ticks had driven my in a flawed learning path.
I wanted the skills needed to create and maintain real, large scale applications. After all, these skills are required to get my Jarvis coded 😜. Competitive Coding would never teach these skills. So after some research, I figured having practical experience with open source programming could be an excellent learning path. In good open source projects you can find well organized and well maintained code for large systems.
I searched everywhere for resources to help me get started with open source. Much to my surprise, I found very little. Ironically, there are lots of in-depth articles to help you get started with Competitive Coding. For those starting with open source, only a few shallow ones. That’s why I decided to record my insights in this article: to help people get started in open source contributing.
Disclaimer: I’m not an experienced open source contributor yet. What I wrote here is a compilation of the advice I got from senior friends and resources I read online. Also, I love competitive coding and I am in no way criticizing it. I’m just trying to say that learning to code via competitive coding alone can be dangerous.
Choose the Right Project
Don’t pick an open source project just for the sake of contributing to open source. That won’t work in the long run. You’ll quickly loose the motivation to keep working on the project. Find a software that you can become a user, or at least a product that you genuinely care about.
To make things easier, start using more open source software regularly. Naturally, you’ll come up with ideas for new features or improvements for the stuff you’re using. That will get you much more excited than randomly hopping in Github projects trying to find some work you can do.
When deciding work on an open source project, check if its level of complexity is in pair with your expertise level. If you decide to work on the Linux Kernel for starters, that’ll probably be a struggle that will hardly get you started. It’s much easier to start working on a small codebase. For instance, you can start with a browser plug-in, or an educational project like TodoMVC.
Become Proficient at Reading Code
Composers listen to a lot of music, before they try creating interesting melodies of their own. Similarly, to become a writer you should read many good books prior to your writing. Novice actors also spend a lot of time observing how experienced actors personify their characters. In coding it’s no different: you learn a lot by reading well-written code authored by others.
Moreover, reading code is an essential skill as you must be able to read and understand a codebase before you can effectively change it. Most of the time you’ll read code rather than write. And that’s true not just for open source. Join any software house, and you’ll get confronted with a huge pile of code you’ll be required to change. The hardest thing in this process is understanding how the existing code works. And the better you are at reading code, the easier it is to change code.
It’s not uncommon to work in projects with thousands of lines of code. In such large projects, its impossible to understand the whole thing at once. As you get used to reading code, you’ll get better at understanding small parts of a very large code base. And as you get used to read code piece-by-piece, it strengthens an important skill: your ability to modularize your own code in easily digestible modules.
But hey, once you decide you’ll work on a project, don’t go read its source code straight away. Do this before you start reading:
- Check if the project’s website or source code includes documentation. If yes, read it first.
- Run/compile the code from its sources. That will greatly help you understand how the app behaves.
- Ask yourself, “how would you have implemented this app?” That’s a good way to start the process of getting your mindset closer to that of the app’s developers.
When you’re ready to explore the source code, you can get started like this:
- Make sense of the project’s file structure. How many files it has? Which are the main ones?
- Understand the naming conventions used in the code. Figure out how the files and folders were named. Get a sense of which file/classs/function name does what.
- Do a quick read. In the process, don’t immediately investigate concepts you’re not familiar with. Keep a list of unknown concepts that you’ll explore later. This keeps you grounded on the source code and avoids distractions.
- Test individual, simple features by running them inside a debugger. First look at the code and try guess of how it works. Then run it through the debugger to see if you were right.
- If you find any part of the code strange or confusing, add a comment to that part, so you can further investigate later.
By repeating this process, you’ll slowly grow your knowledge on how the code works. Things will gradually become clearer.
Also, be aware that some open source projects have a special “annotated” or “light” versions that are much easier to read. For instance, for jQuery there is the amazing “jQuery Annotated Source”. For reactJS there is a lightweight version called “preactJS”, which is like the original but with much less features, so it’s way easier to read.
There are also some books on how famous open source projects are built. For instance, for AngularJS, there is the excellent book “Build your own AngularJS”, that teaches the reader to implement AngularJS piece by piece.
Finally, you might identify yourself with some particular developer’s code, and find it easier to read. So if you find some code you love, check if its developer also published other sources, and read those as well.
Write Tests
Many open source projects include tests. If your proposed code contribution doesn’t include tests, it has a higher probability of not being accepted. So make sure to include tests along with every bit of code you propose adding.
Tests are nothing to be scared of. They’re just some extra code. Think of them as tiny requirements for each added feature. Many people even advocate that you should first write the test for the feature you want to add, and only then write the code that implements said feature.
Some junior programmers might dislike or even hate writing tests. With time, however, every coder comes to appreciate its benefits. Writing tests change the way you write code for the better.
Usually, the code we’re working with is made of smaller pieces. For the whole code to work, each piece must fulfill specific requirements. For small code bases, we can mentally keep track of those requirements as we’re working on it. But as the project grows, that becomes impossible.
Very often, we might change something and unknowingly break a requirement. By running tests, we’re aware if we break something. Tests provide an automated way to quickly verify that every requirement from every code piece is being met. Relying on the tests, we can play with the code and still be certain that it works, as long as our modifications don’t break the tests.
Moreover, tests are also a very valuable resource when reading code, as they also function as an extra documentation layer. They often provide common examples of how to use the app, and extensively list what the app is able to do.
Don’t be scared of Git
Without knowing how to use the Git version control system, you’ll hardly be able to share your open source work with others. You should invest some time in learning the basics of how Git works. Keep in mind that Git is an extremely powerful tool, that offers several ways for things to get done. Learning Git takes a lot of time. You’ll probably keep learning it for as long as you are writing code.
If you’re a complete beginner, try the Github desktop client, as it has a graphical user interface. Afterwards you can gradually switch to the terminal if you want. The graphical client does a good job of showing you what’s happening with your files, even if you know nearly nothing about git commands.
Even with the GUI Git program, many beginners are scared they might break things when using Git. To avoid that stress, start using Git not in a project you care about, but in a playground project. The important thing is to get started.
Create a sample project in Github just for learning and playing with Git. You can get one of your friends to contribute to that dummy project. Have him send you Pull Requests in Github, so you know how the process works. Create different branches, see how they show in Github, and in the Desktop client. Have you and your friend edit the same line in the same file, so you have a feeling of what is a merge conflict and how it gets solved.
Git tutorials explain just the bare basics of Git, but you’ll really only learn to deal with real-word use cases of Git with practise.
Sending Pull Requests
Once you make some code changes to an open source project hosted on Github, you’ll want to send a Pull Request. That’s a way to communicate to the project’s maintainers what you changed—what problems did you solve, and why should they accept your modifications.
When making pull requests, make sure you include a message explaining very clearly what did you change, and why did you change it.
Set your ego aside when sending your pull requests. People are going to criticize you, but they don’t mean to hurt your feelings. If your pull request gets rejected, take the reply as valuable feedback. Make the changes that are being required, and submit it again!
Squash “first-timer” tickets
Lately, many open source projects are making some easy-issues as “first-timers” only. These are the issues dedicated to people who want to make their 1st pull request, and their 1st contribution. If your project offers those, that’s a very good way to get started.
Contribute with Documentation
You can also get involved in a project even if you don’t write any code. If you find a way to better explain a concept in the project’s documentation, go for it! Even if your contribution is just for fixing typos. You can create a pull request that just changes the project’s documentation. Besides playing with a “sandbox” git project, creating pull requests that just change text in a project’s documentation is also a nice way to get confortable with Git and build some confidence. It’s also a great way to start a relationship the project’s maintainers.